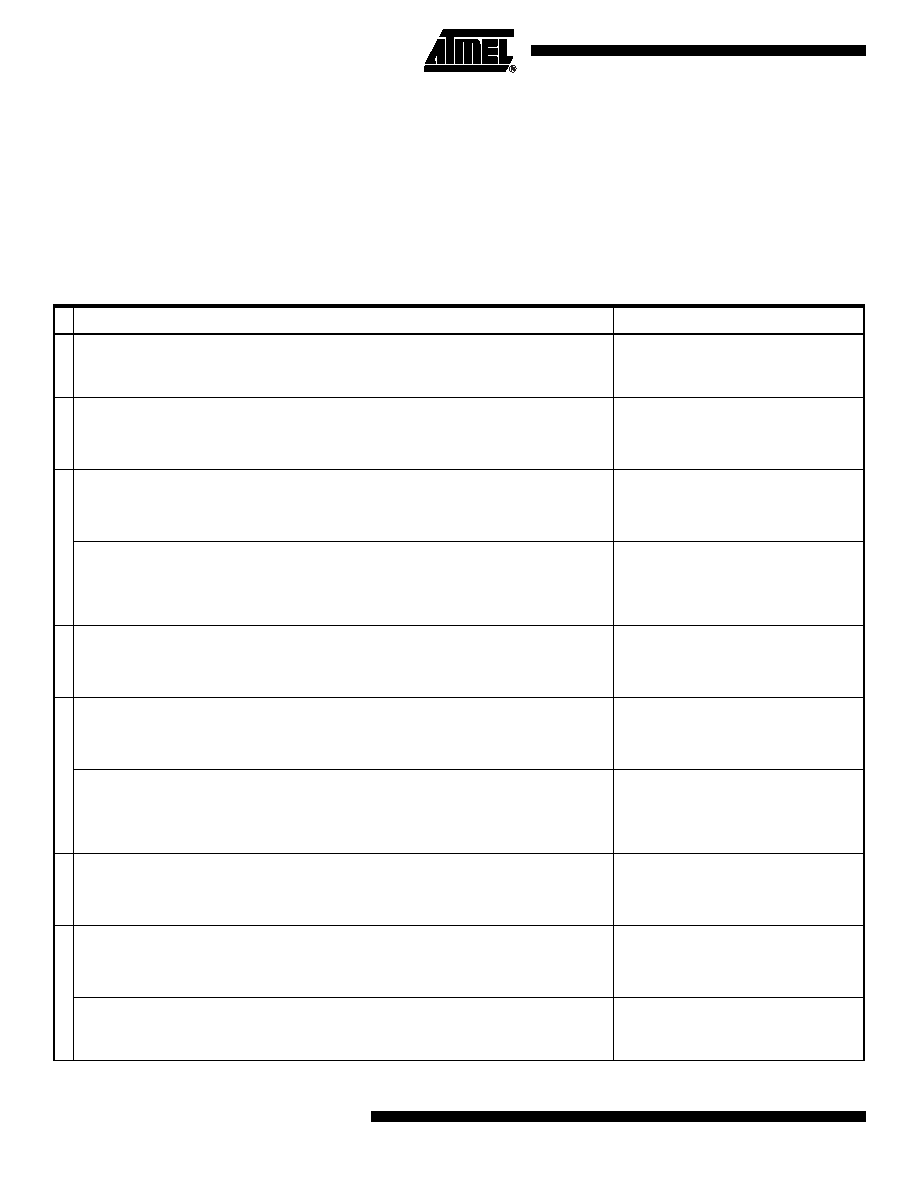
216
7682C–AUTO–04/08
AT90CAN32/64/128
When the TWINT flag is set, the user must update all TWI Registers with the value relevant
for the next TWI bus cycle. As an example, TWDR must be loaded with the value to be
transmitted in the next bus cycle.
After all TWI Register updates and other pending application software tasks have been
completed, TWCR is written. When writing TWCR, the TWINT bit should be set. Writing a
one to TWINT clears the flag. The TWI will then commence executing whatever operation
was specified by the TWCR setting.
In the following an assembly and C implementation of the example is given. Note that the code
below assumes that several definitions have been made for example by using include-files.
Assembly Code Example
C Example
Comments
1
ldi
r16, (1<<TWINT)|
(1<<TWSTA)|
(1<<TWEN)
sts
TWCR, r16
TWCR = (1<<TWINT)|
(1<<TWSTA)|
(1<<TWEN)
Send START condition
2
wait1:
lds
r16,TWCR
sbrs
r16,TWINT
rjmp
wait1
while (!(TWCR & (1<<TWINT)));
Wait for TWINT flag set. This indicates that
the START condition has been transmitted
3
lds
r16,TWSR
andi
r16, 0xF8
cpi
r16, START
brne
ERROR
if ((TWSR & 0xF8)!= START)
ERROR();
Check value of TWI Status Register. Mask
prescaler bits. If status different from START
go to ERROR
ldi
r16, SLA_W
sts
TWDR, r16
ldi
r16, (1<<TWINT)|
(1<<TWEN)
sts
TWCR, r16
TWDR = SLA_W;
TWCR = (1<<TWINT)|(1<<TWEN);
Load SLA_W into TWDR Register. Clear
TWINT bit in TWCR to start transmission of
address
4
wait2:
lds
r16,TWCR
sbrs
r16,TWINT
rjmp
wait2
while (!(TWCR & (1<<TWINT)));
Wait for TWINT flag set. This indicates that
the SLA+W has been transmitted, and
ACK/NACK has been received.
5
lds
r16,TWSR
andi
r16, 0xF8
cpi
r16, MT_SLA_ACK
brne
ERROR
if ((TWSR & 0xF8)!= MT_SLA_ACK)
ERROR();
Check value of TWI Status Register. Mask
prescaler bits. If status different from
MT_SLA_ACK go to ERROR
ldi
r16, DATA
sts
TWDR, r16
ldi
r16, (1<<TWINT)|
(1<<TWEN)
sts
TWCR, r16
TWDR = DATA;
TWCR = (1<<TWINT)|(1<<TWEN);
Load DATA into TWDR Register. Clear TWINT
bit in TWCR to start transmission of data
6
wait3:
lds
r16,TWCR
sbrs
r16,TWINT
rjmp
wait3
while (!(TWCR & (1<<TWINT)));
Wait for TWINT flag set. This indicates that
the DATA has been transmitted, and
ACK/NACK has been received.
7
lds
r16,TWSR
andi
r16, 0xF8
cpi
r16, MT_DATA_ACK
brne
ERROR
if ((TWSR & 0xF8)!=MT_DATA_ACK)
ERROR();
Check value of TWI Status Register. Mask
prescaler bits. If status different from
MT_DATA_ACK go to ERROR
ldi
r16, (1<<TWINT)|
(1<<TWEN) |
(1<<TWSTO)
sts
TWCR, r16
TWCR = (1<<TWINT)|
(1<<TWEN) |
(1<<TWSTO);
Transmit STOP condition