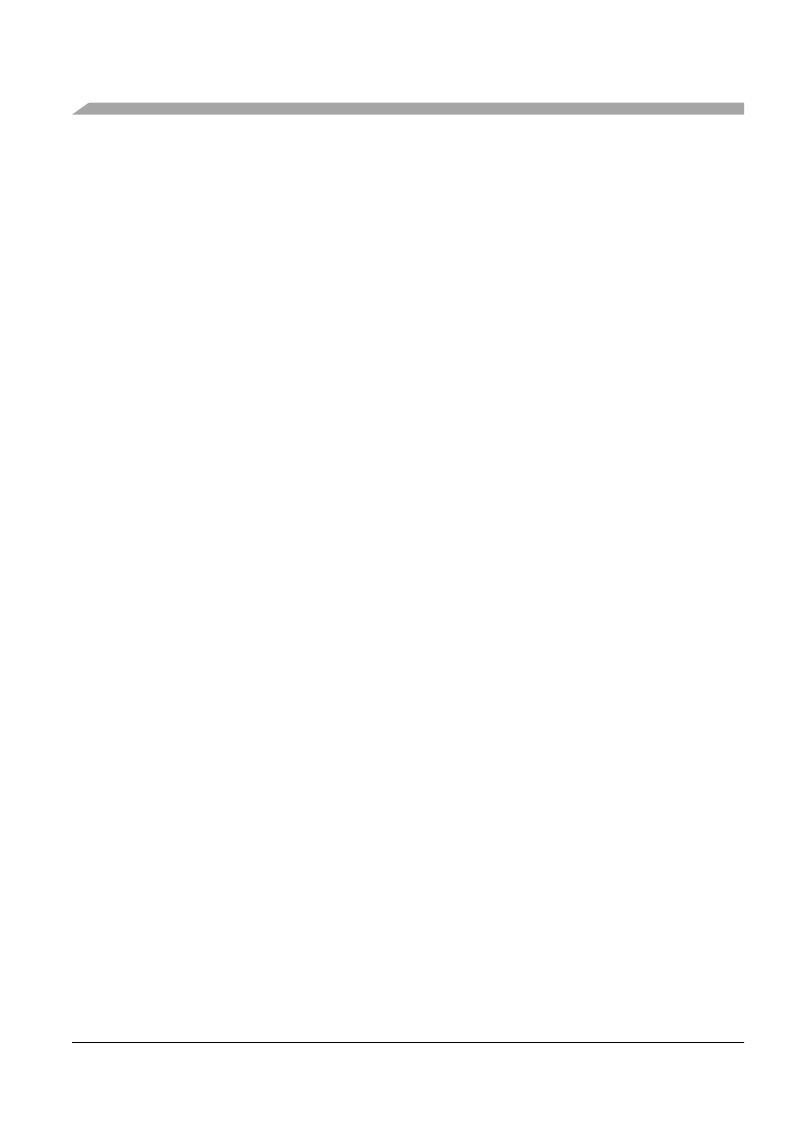
On-Chip FLASH Programming Routines, Rev. 4
28
Freescale Semiconductor
On-Chip Routines Source Code
;* VARIABLES MODIFIED:
;* STACK USED: 4 (including the call to this routine)
;* SIZE: 31 bytes
;* DESCRIPTION: EXECUTED OUT OF ROM
;* Execution cycle for Internal and external is:
;* Internal (OSCSTAT[0]=0) = 28 + (10 x SampPerBitI)
;* Extnernal (OSCSTAT[0]=1) = 27 + (10 x SampPerBitE)
;*********************************************************************
GetBit: pshx ;[2] preserve X
psha ;[2] preserve A
nop ;[1] time padding
brset 0,OSCSTAT,SerialE ;[5] check if int or ext clk
lda #OffsetI ;[2] # of samples to detect 1 (Int)
ldx #SampPerBitI ;[2] # of samples per bit (Int)
brclr 0,OSCSTAT,loopIn ;[5] time matching padding
SerialE: lda #OffsetE ;[2] # of samples to detect 1 (Ext)
ldx #SampPerBitE ;[2] # of samples per bit (Ext)
nop ;[1] time padding
bra loopIn ;[3] time padding
loopIn: brclr 0,PTA,subSamp ;[5] set/clr C based on PTA0 level
subSamp: sbc #0 ;[2] subtract C from offset in A
dbnzx loopIn ;[3] loop SampPerBitI times
rola ;[1] copy MSB to C bit (1 if A neg)
;* A would be negative if # of 1 samples was > OffsetG_
;* C bit reflects detected sense of current serial bit
pula ;[2] restore A
pulx ;[2] restore X
rts ;[4] return
;* GetBit DONE ******************
;*********************************************************************
;* NAME: RDVRRNG
;* PURPOSE: Read and/or verify a range of FLASH memory
;* ENTRY CONDITIONS:
;* H:X contains a start address of the FLASH address range
;* LADDR:LADDR+1 contains a last address of the FLASH address range
;* The contents of A decides if read data is transferred serially
;* via PTA0 (When A=0, PTA0 is used for serial transfer) or
;* the data is verified against the DATA array in RAM
;* DATA array contains the data to be verified
;* If A=0, PTA0 is configured as an input (DDRA0=0) and
;* data bit = 0 (PTA0=0)
;* EXIT CONDITIONS:
;* A contains checksum
;* C-bit in CCR indicates verify result when entry A is NOT zero
;* If C-bit is set, the verify is successful
;* DATA array contains read FLASH data when entry A is NOT zero
;* H:X contains a next FLASH read address
;* SUBROUTINES CALLED: PutByte
;* VARIABLES READ: LADDR:LADDR+1,DATA array
;* VARIABLES MODIFIED: DATA array
;* STACK USED: (include the call to this routine)
;* 9 bytes for Verify operation (entry A is NOT zero)
;* 11 bytes for data send out operation (entry A is zero)
;* SIZE: 67 bytes
;* DESCRIPTION: Executed out of ROM
;* The COP is serviced in this routine. The first COP is serviced on